Using Lookup Tables
Introduction
In this tutorial we will revisit Constant tables and use one of its very useful functionalities: lookup
.
Lookup tables allow you to implement something called Decision tables. This should help simplify the logic we've written in the previous tutorial where we calculated the age surcharge.
We'll be rewriting code in this tutorial, but the overall logic should not change. Our Testcase should still succeed after our changes!
Task: Create the lookup table
Open the
Travel insurance calculator
from the previous tutorials.Let's first delete these three constants:
ExtraCostPerDay18_30
ExtraCostPerDay31_50
ExtraCostPerDay51AndUp
Note: you can do this by clicking the (...) icon of the constant in the menu and clicking Delete OR opening the constant and clicking "Delete" in the bottom right corner of the screen.
Create a new constant named
ExtraCostsPerDay
and set its Constant Type to Table
You should be somewhat familiar with Constant tables if you've followed the tutorials in order so far. Let's start filling the table with data.
First, click the + COLUMN button to add a new column
Rename the
value
column toage
Rename the
value2
column toresult
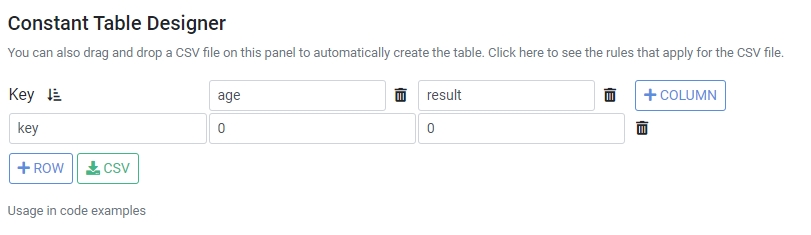
Keys are not as important in lookup tables, we will start with with 1 and let it automatically increment.
Click on the first cell (key)
Enter
1
in this cellPress Tab to move to the
age
columnEnter
< 18
and press TabLeave the result cell
0
and press Enter
This should create a new row with a key that is 2
.
Enter the following rows to complete the table:
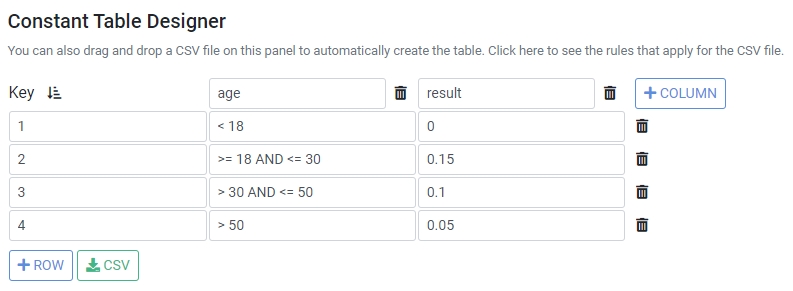
Alternatively, copy and paste the following CSV while you have the ExtraCostsPerDay
constant open.
key,age,result
1,< 18,0
2,>= 18 AND <= 30,0.15
3,> 30 AND <= 50,0.1
4,> 50,0.05
Note: Make sure you are not in a text field when you paste, or the contents of the CSV will be pasted in that instead.
How does it work?
You may have noticed that the cells under the age column are expressions and not plain values. These expressions are used when the lookup()
method is used.
For example you could try this in a new rule named LookupTest
:
ExtraCostsPerDay.lookup(20)
This will look up the value 20
in the table in the first column (the Key column is not counted) and return the value in the last column. This will result in 0.15
, because the second row's expression (>= 18 AND <= 30
) satisfies for the given input of 20
.
Task: Simplify the AgeSurcharge
function
AgeSurcharge
functionNext we're going to simplify the AgeSurcharge
function. Its Function body currently should look something like this:
if (age >= 18 && age < 31) {
return numberOfDays * ExtraCostPerDay18_30;
}
if (age >= 31 && age < 51) {
return numberOfDays * ExtraCostPerDay31_50;
}
if (age >= 51) {
return numberOfDays * ExtraCostPerDay51AndUp;
}
return 0;
We're going to actually replace this with just a single line of code!
Delete the contents of
AgeSurcharge
's Function body codeAdd the following code:
return numberOfDays * ExtraCostsPerDay.lookup(age);
Save the ruleset
If everything went well, the ruleset should work as before. If you followed the previous tutorial on adding Testcases, the Testcase we've created should still succeed, as we did not change the overall logic.
Recap
Complex code that deals with calculations with tiered values can be rewritten using Constant tables and their lookup()
method.
This reduces code in your ruleset and allows for easy management of business logic.